今回は指定したオブジェクトをランダムに配置するシステムをつくってみたので紹介していきます。
完成イメージ
実行を押すと…
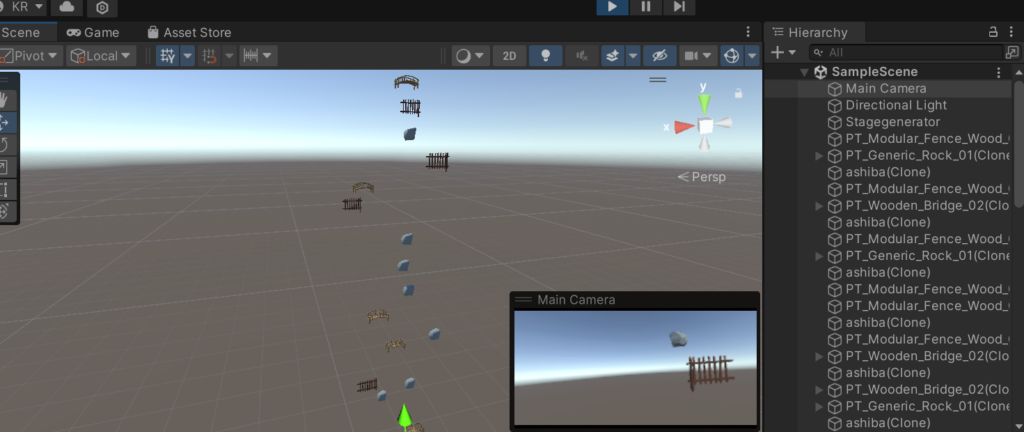
このようにオブジェクトの種類や、範囲、感覚などを指定することができます。今回はマインクラフトのThe Dropperを意識して作ったので、同時にplayerとその足場も生成できるようにしました。
つくり方
やり方は非常に簡単です。まずは
[Hierarchy]内に空のオブジェクトを作り、「Stagegenerator」と名付けます。
これに次のコードをアタッチするだけです。
using UnityEngine;
public class DropperMapGenerator : MonoBehaviour
{
public GameObject playerPrefab;
public GameObject[] obstaclePrefabs;
public GameObject platformPrefab;
public float mapLength = 100f;
public int numSections = 20; //障害物の数
public float sectionHeight = 5f;
public float sectionWidth = 10f;
[Range(0f, 1f)]
public float obstacleSpacing = 0.5f; // 障害物の間隔 (0~1の範囲で指定)。小さいほど密集。
private GameObject player; //playerを生成する必要がないときは削除してください
private float maxObstacleHeight;
void Start()
{
GenerateMap();
SpawnPlayer();
}
void GenerateMap()
{
maxObstacleHeight = 0f;
for (int i = 0; i < numSections; i++)
{
float sectionStart = i * (mapLength / numSections);
float sectionEnd = sectionStart + (mapLength / numSections);
float obstacleSpan = (mapLength / numSections) * obstacleSpacing; // 障害物の間隔
float nextObstaclePosition = sectionStart;
while (nextObstaclePosition < sectionEnd)
{
Vector3 position = new Vector3(
Random.Range(-sectionWidth / 2f, sectionWidth / 2f),
nextObstaclePosition,
Random.Range(-sectionWidth / 2f, sectionWidth / 2f)
);
GameObject obstaclePrefab = obstaclePrefabs[Random.Range(0, obstaclePrefabs.Length)];
GameObject obstacle = Instantiate(obstaclePrefab, position, Quaternion.identity);
maxObstacleHeight = Mathf.Max(maxObstacleHeight, obstacle.transform.position.y);
nextObstaclePosition += obstacleSpan;
}
Vector3 platformPosition = new Vector3(0f, sectionStart, 0f);
Instantiate(platformPrefab, platformPosition, Quaternion.identity);
}
}
//キャラクターを生成する必要がないのであれば削除してください。
void SpawnPlayer()
{
player = Instantiate(playerPrefab, new Vector3(0f, maxObstacleHeight + 5f, 0f), Quaternion.identity);
}
}
これで完成です。注意点は、Prefab化する前にオブジェクトたちはcollider設定やらrigidboyやらをつけ忘れない様にするってところ。
あとデメリットを挙げるとしたら、毎回自動生成なのでステージが変わっちゃうという点。
でもランダム生成のオブジェクト配置とか、範囲指定してランダムに物を隠すときとかに流用できそうです。
コメントを残す コメントをキャンセル